Labware¶
Labware are the durable or consumable items that you work with, reuse, or discard while running a protocol on a Flex or OT-2. Items such as pipette tips, well plates, tubes, and reservoirs are all examples of labware. This section provides a brief overview of default labware, custom labware, and how to use basic labware API methods when creating a protocol for your robot.
Note
Code snippets use coordinate deck slot locations (e.g. "D1"
, "D2"
), like those found on Flex. If you have an OT-2 and are using API version 2.14 or earlier, replace the coordinate with its numeric OT-2 equivalent. For example, slot D1 on Flex corresponds to slot 1 on an OT-2. See Deck Slots for more information.
Labware Types¶
Default Labware¶
Default labware is everything listed in the Opentrons Labware Library. When used in a protocol, your Flex or OT-2 knows how to work with default labware. However, you must first inform the API about the labware you will place on the robot’s deck. Search the library when you’re looking for the API load names of the labware you want to use. You can copy the load names from the library and pass them to the load_labware()
method in your protocol.
Custom Labware¶
Custom labware is labware that is not listed the Labware Library. If your protocol needs something that’s not in the library, you can create it with the Opentrons Labware Creator. However, before using the Labware Creator, you should take a moment to review the support article Creating Custom Labware Definitions.
After you’ve created your labware, save it as a .json
file and add it to the Opentrons App. See Using Labware in Your Protocols for instructions.
If other people need to use your custom labware definition, they must also add it to their Opentrons App.
Loading Labware¶
Throughout this section, we’ll use the labware listed in the following table.
Labware type |
Labware name |
API load name |
---|---|---|
Well plate |
|
|
Flex tip rack |
|
|
OT-2 tip rack |
|
Similar to the code sample in How the API Works, here’s how you use the ProtocolContext.load_labware()
method to load labware on either Flex or OT-2.
#Flex
tiprack = protocol.load_labware("opentrons_flex_96_tiprack_200ul", "D1")
plate = protocol.load_labware("opentrons_96_wellplate_200ul_pcr_full_skirt", "D2")
#OT-2
tiprack = protocol.load_labware("opentrons_96_tiprack_300ul", "1")
plate = protocol.load_labware("opentrons_96_wellplate_200ul_pcr_full_skirt", "2")
New in version 2.0.
When the load_labware
method loads labware into your protocol, it returns a Labware
object.
Tip
The load_labware
method includes an optional label
argument. You can use it to identify labware with a descriptive name. If used, the label value is displayed in the Opentrons App. For example:
tiprack = protocol.load_labware(
load_name="opentrons_flex_96_tiprack_200ul",
location="D1",
label="any-name-you-want")
Loading Lids¶
You can load lids on compatible plates or tip racks with the optional lid
parameter of load_labware()
. This example adds an Opentrons Tough Auto-Sealing Lid to a PCR plate:
plate = protocol.load_labware(
load_name="opentrons_96_wellplate_200ul_pcr_full_skirt",
location="D2",
lid="opentrons_tough_pcr_auto_sealing_lid"
)
And this example loads an Opentrons Flex Tip Rack Lid onto a rack of 200 µL tips:
tiprack = protocol.load_labware(
load_name="opentrons_flex_96_tiprack_200ul",
location="D1",
lid="opentrons_flex_tiprack_lid"
)
You might need multiple lids during your protocol. Use ProtocolContext.load_lid_stack()
to stack up to five Opentrons Tough Auto-Sealing Lids on a deck slot, or use Labware.load_lid_stack()
to stack them on the riser or other compatible adapter.
lid_stack = protocol.load_lid_stack(
load_name="opentrons_tough_pcr_auto_sealing_lid",
location="B2",
quantity=4
)
Tip rack lids can’t be stacked or placed on the deck.
New in version 2.23.
Loading Labware on Adapters¶
The previous section demonstrates loading labware directly into a deck slot. But you can also load labware on top of an adapter that either fits on a module or goes directly on the deck. The ability to combine labware with adapters adds functionality and flexibility to your robot and protocols.
You can either load the adapter first and the labware second, or load both the adapter and labware all at once.
Loading Separately¶
The load_adapter()
method is available on ProtocolContext
and module contexts. It behaves similarly to load_labware()
, requiring the load name and location for the desired adapter. Load a module, adapter, and labware with separate calls to specify each layer of the physical stack of components individually:
hs_mod = protocol.load_module("heaterShakerModuleV1", "D1")
hs_adapter = hs_mod.load_adapter("opentrons_96_flat_bottom_adapter")
hs_plate = hs_adapter.load_labware("nest_96_wellplate_200ul_flat")
New in version 2.15: The load_adapter()
method.
Loading Together¶
Use the adapter
argument of load_labware()
to load an adapter at the same time as labware. For example, to load the same 96-well plate and adapter from the previous section at once:
hs_plate = hs_mod.load_labware(
name="nest_96_wellplate_200ul_flat",
adapter="opentrons_96_flat_bottom_adapter"
)
New in version 2.15: The adapter
parameter.
Note
The API also has some “combination” labware definitions, which treat the adapter and labware as a unit:
hs_combo = hs_mod.load_labware(
"opentrons_96_flat_bottom_adapter_nest_wellplate_200ul_flat"
)
Deprecated since version 2.15.
Labware loaded with combination adapters no longer support all API features, such as moving the top labware or pipetting relative to liquid meniscus. These definitions are marked as “Retired” in version 8.5.0 of the Opentrons App and later. Avoid using combination definitions unless your protocol specifies an apiLevel
of 2.14 or lower, in which case they are required.
Accessing Wells in Labware¶
Well Ordering¶
You need to select which wells to transfer liquids to and from over the course of a protocol.
Rows of wells on a labware have labels that are capital letters starting with A. For instance, an 96-well plate has 8 rows, labeled "A"
through "H"
.
Columns of wells on a labware have labels that are numbers starting with 1. For instance, a 96-well plate has columns "1"
through "12"
.
All well-accessing functions start with the well at the top left corner of the labware. The ending well is in the bottom right. The order of travel from top left to bottom right depends on which function you use.
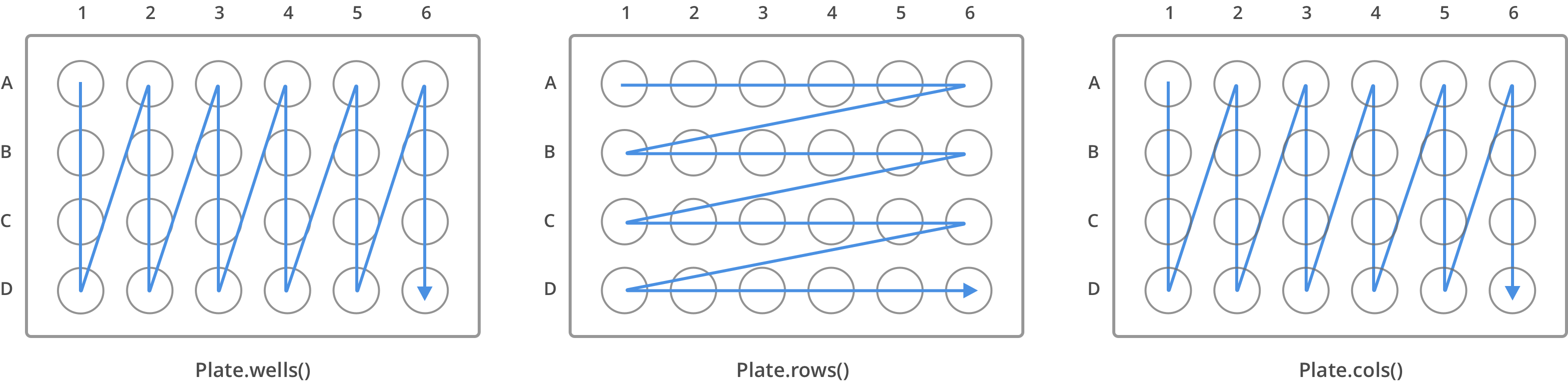
The code in this section assumes that plate
is a 24-well plate. For example:
plate = protocol.load_labware("corning_24_wellplate_3.4ml_flat", location="D1")
Accessor Methods¶
The API provides many different ways to access wells inside labware. Different methods are useful in different contexts. The table below lists out the methods available to access wells and their differences.
Method |
Returns |
Example |
---|---|---|
List of all wells. |
|
|
List of lists grouped by row. |
|
|
List of lists grouped by column. |
|
|
Dictionary with well names as keys. |
|
|
Dictionary with row names as keys. |
|
|
Dictionary with column names as keys. |
|
Accessing Individual Wells¶
Dictionary Access¶
The simplest way to refer to a single well is by its well_name
, like A1 or D6. Referencing a particular key in the result of Labware.wells_by_name()
accomplishes this. This is such a common task that the API also has an equivalent shortcut: dictionary indexing.
a1 = plate.wells_by_name()["A1"]
d6 = plate["D6"] # dictionary indexing
If a well does not exist in the labware, such as plate["H12"]
on a 24-well plate, the API will raise a KeyError
. In contrast, it would be a valid reference on a standard 96-well plate.
New in version 2.0.
List Access From wells
¶
In addition to referencing wells by name, you can also reference them with zero-indexing. The first well in a labware is at position 0.
plate.wells()[0] # well A1
plate.wells()[23] # well D6
Tip
You may find coordinate well names like "B3"
easier to reason with, especially when working with irregular labware, e.g.
opentrons_10_tuberack_falcon_4x50ml_6x15ml_conical
(see the Opentrons 10 Tube Rack in the Labware Library). Whichever well access method you use, your protocol will be most maintainable if you use only one access method consistently.
New in version 2.0.
Accessing Groups of Wells¶
When handling liquid, you can provide a group of wells as the source or destination. Alternatively, you can take a group of wells and loop (or iterate) through them, with each liquid-handling command inside the loop accessing the loop index.
Use Labware.rows_by_name()
to access a specific row of wells or Labware.columns_by_name()
to access a specific column of wells on a labware. These methods both return a dictionary with the row or column name as the keys:
row_dict = plate.rows_by_name()["A"]
row_list = plate.rows()[0] # equivalent to the line above
column_dict = plate.columns_by_name()["1"]
column_list = plate.columns()[0] # equivalent to the line above
print('Column "1" has', len(column_dict), 'wells') # Column "1" has 4 wells
print('Row "A" has', len(row_dict), 'wells') # Row "A" has 6 wells
Since these methods return either lists or dictionaries, you can iterate through them as you would regular Python data structures.
For example, to transfer 50 µL of liquid from the first well of a reservoir to each of the wells of row "A"
on a plate:
for well in plate.rows()[0]:
pipette.transfer(reservoir["A1"], well, 50)
Equivalently, using rows_by_name
:
for well in plate.rows_by_name()["A"].values():
pipette.transfer(reservoir["A1"], well, 50)
New in version 2.0.
Labeling Liquids in Labware¶
Optionally, you can specify the liquids that should be in labware at the beginning of your protocol. Doing so helps you identify well contents by name and volume, and adds corresponding labels to a single well, group of wells, or an entire labware. You can view the initial liquid setup:
For Flex protocols, on the touchscreen.
For Flex or OT-2 protocols, in the Opentrons App (v6.3.0 or higher).
To use these optional methods, first create a liquid object with ProtocolContext.define_liquid()
and then label individual wells by calling Labware.load_liquid()
.
Let’s examine how these two methods work. The following examples demonstrate how to define colored water samples for a well plate and reservoir.
Defining Liquids¶
This example uses define_liquid
to create two liquid objects and instantiates them with the variables greenWater
and blueWater
, respectively. The arguments for define_liquid
are all required, and let you name the liquid, describe it, and assign it a color:
greenWater = protocol.define_liquid(
name="Green water",
description="Green colored water for demo",
display_color="#00FF00",
)
blueWater = protocol.define_liquid(
name="Blue water",
description="Blue colored water for demo",
display_color="#0000FF",
)
New in version 2.14.
The display_color
parameter accepts a hex color code, which adds a color to that liquid’s label when you import your protocol into the Opentrons App. The define_liquid
method accepts standard 3-, 4-, 6-, and 8-character hex color codes.
Labeling Wells and Reservoirs¶
This example uses load_liquid
to label the initial well location, contents, and volume (in µL) for the liquid objects created by define_liquid
. Notice how values of the liquid
argument use the variable names greenWater
and blueWater
(defined above) to associate wells in each labware with a particular liquid:
## load entire well plate with greenWater
plate.load_liquid(
wells=plate.wells(), # using accessor
volume=50,
liquid=greenWater
)
## load entire reservoir with blueWater
reservoir.load_liquid(
wells=[A1], # using list of well names
volume=50,
liquid=blueWater
)
load_liquid
makes it easy to load an entire well plate with a single command. Let’s say you only need to load liquid in a few wells, or want to load multiple liquids or volumes in the same labware.
plate.load_liquid_by_well({'A1': 200, 'A2': 100, 'A3': 50}, greenWater)
plate.load_liquid_by_well({'B1': 200, 'B2': 100, 'B3': 50}, blueWater)
You can also use Labware.load_empty()
to label individual wells or an entire labware as empty at the beginning of your protocol.
New in version 2.14: Use Well.load_liquid()
to label liquid in individual wells.
New in version 2.22: Use Labware.load_liquid
, Labware.load_liquid_by_well
, or Labware.load_empty
to label liquid in individual wells or an entire labware.
This information is available after you import your protocol to the app or send it to Flex. A summary of liquids appears on the protocol detail page, and well-by-well detail is available on the run setup page (under Initial Liquid Setup in the app, or under Liquids on Flex).
Note
load_liquid
does not validate volume for your labware nor does it prevent you from adding multiple liquids to each well. For example, you could label a 40 µL well with greenWater
, volume=50
, and then also add blue water to the well. The API won’t stop you. It’s your responsibility to ensure the labels you use accurately reflect the amounts and types of liquid you plan to place into wells and reservoirs.
Labeling vs Handling Liquids¶
The load_liquid
arguments include a volume amount (volume=n
in µL). This amount is just a label. It isn’t a command or function that manipulates liquids. It only tells you how much liquid should be in a well at the start of the protocol. You need to use a method like transfer()
to physically move liquids from a source to a destination.
Although it’s optional to define and load liquids in your protocol, you can use a starting liquid volume to specify pipette movements relative to a liquid location, like the meniscus, in your protocol.
Well Dimensions¶
The functions in the Accessing Wells in Labware section above return a single Well
object or a larger object representing many wells. Well
objects have attributes that provide information about their physical shape, such as the depth or diameter, as specified in their corresponding labware definition. These properties can be used for different applications, such as calculating the volume of a well or a position relative to the well.
Depth¶
Use Well.depth
to get the distance in mm between the very top of the well and the very bottom. For example, a conical well’s depth is measured from the top center to the bottom center of the well.
plate = protocol.load_labware("corning_96_wellplate_360ul_flat", "D1")
depth = plate["A1"].depth # 10.67
Diameter¶
Use Well.diameter
to get the diameter of a given well in mm. Since diameter is a circular measurement, this attribute is only present on labware with circular wells. If the well is not circular, the value will be None
. Use length and width (see below) for non-circular wells.
plate = protocol.load_labware("corning_96_wellplate_360ul_flat", "D1")
diameter = plate["A1"].diameter # 6.86
Length¶
Use Well.length
to get the length of a given well in mm. Length is defined as the distance along the robot’s x-axis (left to right). This attribute is only present on rectangular wells. If the well is not rectangular, the value will be None
. Use diameter (see above) for circular wells.
plate = protocol.load_labware("nest_12_reservoir_15ml", "D1")
length = plate["A1"].length # 8.2
Width¶
Use Well.width
to get the width of a given well in mm. Width is defined as the distance along the y-axis (front to back). This attribute is only present on rectangular wells. If the well is not rectangular, the value will be None
. Use diameter (see above) for circular wells.
plate = protocol.load_labware("nest_12_reservoir_15ml", "D1")
width = plate["A1"].width # 71.2
New in version 2.9.